本文介绍了Support V4包中DialogFragment、Fragment的Arguments、Fragment的上下文菜单、Fragment的自定义动画、Fragment数据的保存及恢复、Fragment横竖屏的不同布局的实现、ListFragment、创建菜单、嵌套的Tab、Fragment和ViewPager配合使用、Fragment中接收一个结果、Fragment横竖屏切换保持实例、Fragment栈、FragmentStatePagerAdapter及TabHost和ViewPager配合使用。
Alert Dialog
演示了怎样显示一个通过Fragment管理的AlertDialog。
1 | void showDialog() { |
Arguments
演示了一个可以通过Bundle参数和layout属性配置的Fragment。
通过Bundle传参1
Fragment newFragment = MyFragment.newInstance("From Arguments");
通过layout属性配置1
2
3
4
5<fragment class="com.example.android.supportv4.app.FragmentArgumentsSupport$MyFragment"
android:id="@+id/embedded"
android:layout_width="0px" android:layout_height="wrap_content"
android:layout_weight="1"
android:label="@string/fragment_arguments_embedded" />
Fragment
1 | public static class MyFragment extends Fragment { |
Context Menu
演示了从Fragment中显示一个上下文菜单。
1 | public static class ContextMenuFragment extends Fragment { |
Custom Animation
给Fragment设置自定义动画。
1 | // Instantiate a new fragment. |
Dialog
使用DialogFragment显示一个对话框。
Dialog or Activity
演示了同一个Fragment作为dialog显示和嵌入到activity中。
1 | if (savedInstanceState == null) { |
Hide and Show
演示了隐藏和显示fragment并且可以保存和恢复文本数据。第一种使用了Bundle进行数据的保存和恢复,第二种使用了View.setSaveEnabled进行数据的保存和恢复。
1 | void addShowHideListener(int buttonId, final Fragment fragment) { |
Layout
演示了使用fragment实现不同的activity布局。这个例子提供了不同的布局(activity流)当运行在横屏时。
本例子提供了两个不同的布局:横屏和竖屏,竖屏中包含TitlesFragment,横屏中包含TitlesFragment和DetailsFragment。
1 | public class FragmentLayoutSupport extends FragmentActivity { |
竖屏
横屏
List Array
演示了使用ListFragment从数组中显式一个items列表。和上面竖屏效果一样。
1 | public static class ArrayListFragment extends ListFragment { |
Menu
演示了Fragment怎样加入到选项菜单中。1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
/**
* A fragment that displays a menu. This fragment happens to not
* have a UI (it does not implement onCreateView), but it could also
* have one if it wanted.
*/
public static class MenuFragment extends Fragment {
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setHasOptionsMenu(true);
}
@Override
public void onCreateOptionsMenu(Menu menu, MenuInflater inflater) {
MenuItem item;
item = menu.add("Menu 1a");
MenuItemCompat.setShowAsAction(item, MenuItemCompat.SHOW_AS_ACTION_IF_ROOM);
item = menu.add("Menu 1b");
MenuItemCompat.setShowAsAction(item, MenuItemCompat.SHOW_AS_ACTION_IF_ROOM);
}
}
/**
* Second fragment with a menu.
*/
public static class Menu2Fragment extends Fragment {
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setHasOptionsMenu(true);
}
@Override
public void onCreateOptionsMenu(Menu menu, MenuInflater inflater) {
MenuItem item;
item = menu.add("Menu 2");
MenuItemCompat.setShowAsAction(item, MenuItemCompat.SHOW_AS_ACTION_IF_ROOM);
}
}
Nesting Tabs
演示了嵌套的Tab。
1 | public class FragmentNestingTabsSupport extends FragmentActivity { |
Pager
演示了Fragment和ViewPager配合使用。
1 |
|
Receive Result
演示了在Fragment中接收一个结果。
1 | public static class ReceiveResultFragment extends Fragment { |
Retain Instance
这个例子说明了当Activity需要重启时,例如,配置改变时,你可以怎样使用Fragment跨Activity实例方便地传播状态(例如线程)。这个要比使用Activity.onRetainNonConfiguratinInstance() API简单的多。
1 |
|
Stack
演示了Fragment栈(Stack)的使用。
1 |
|
State Pager
演示了记录Fragment状态的Pager,关键类为MyAdapter继承了FragmentStatePagerAdapter。
1 |
|
Tabs
演示了怎样使用FragmentTabHost实现TabHost的tabs之间的切换。
Tabs and Pager
演示了TabHost和ViewPager配合使用实现点击在tabs之间切换并且也允许用户在水平滑动切换tabs。1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
87
88
89
90
91
92
93
94
95
96
97
98
99
100
101
102
103
104
105/**
* This is a helper class that implements the management of tabs and all
* details of connecting a ViewPager with associated TabHost. It relies on a
* trick. Normally a tab host has a simple API for supplying a View or
* Intent that each tab will show. This is not sufficient for switching
* between pages. So instead we make the content part of the tab host
* 0dp high (it is not shown) and the TabsAdapter supplies its own dummy
* view to show as the tab content. It listens to changes in tabs, and takes
* care of switch to the correct paged in the ViewPager whenever the selected
* tab changes.
*/
public static class TabsAdapter extends FragmentPagerAdapter
implements TabHost.OnTabChangeListener, ViewPager.OnPageChangeListener {
private final Context mContext;
private final TabHost mTabHost;
private final ViewPager mViewPager;
private final ArrayList<TabInfo> mTabs = new ArrayList<TabInfo>();
static final class TabInfo {
private final String tag;
private final Class<?> clss;
private final Bundle args;
TabInfo(String _tag, Class<?> _class, Bundle _args) {
tag = _tag;
clss = _class;
args = _args;
}
}
static class DummyTabFactory implements TabHost.TabContentFactory {
private final Context mContext;
public DummyTabFactory(Context context) {
mContext = context;
}
@Override
public View createTabContent(String tag) {
View v = new View(mContext);
v.setMinimumWidth(0);
v.setMinimumHeight(0);
return v;
}
}
public TabsAdapter(FragmentActivity activity, TabHost tabHost, ViewPager pager) {
super(activity.getSupportFragmentManager());
mContext = activity;
mTabHost = tabHost;
mViewPager = pager;
mTabHost.setOnTabChangedListener(this);
mViewPager.setAdapter(this);
mViewPager.setOnPageChangeListener(this);
}
public void addTab(TabHost.TabSpec tabSpec, Class<?> clss, Bundle args) {
tabSpec.setContent(new DummyTabFactory(mContext));
String tag = tabSpec.getTag();
TabInfo info = new TabInfo(tag, clss, args);
mTabs.add(info);
mTabHost.addTab(tabSpec);
notifyDataSetChanged();
}
@Override
public int getCount() {
return mTabs.size();
}
@Override
public Fragment getItem(int position) {
TabInfo info = mTabs.get(position);
return Fragment.instantiate(mContext, info.clss.getName(), info.args);
}
@Override
public void onTabChanged(String tabId) {
int position = mTabHost.getCurrentTab();
mViewPager.setCurrentItem(position);
}
@Override
public void onPageScrolled(int position, float positionOffset, int positionOffsetPixels) {
}
@Override
public void onPageSelected(int position) {
// Unfortunately when TabHost changes the current tab, it kindly
// also takes care of putting focus on it when not in touch mode.
// The jerk.
// This hack tries to prevent this from pulling focus out of our
// ViewPager.
TabWidget widget = mTabHost.getTabWidget();
int oldFocusability = widget.getDescendantFocusability();
widget.setDescendantFocusability(ViewGroup.FOCUS_BLOCK_DESCENDANTS);
mTabHost.setCurrentTab(position);
widget.setDescendantFocusability(oldFocusability);
}
@Override
public void onPageScrollStateChanged(int state) {
}
}
从Android SDK目录可找到源码,或从这里下载
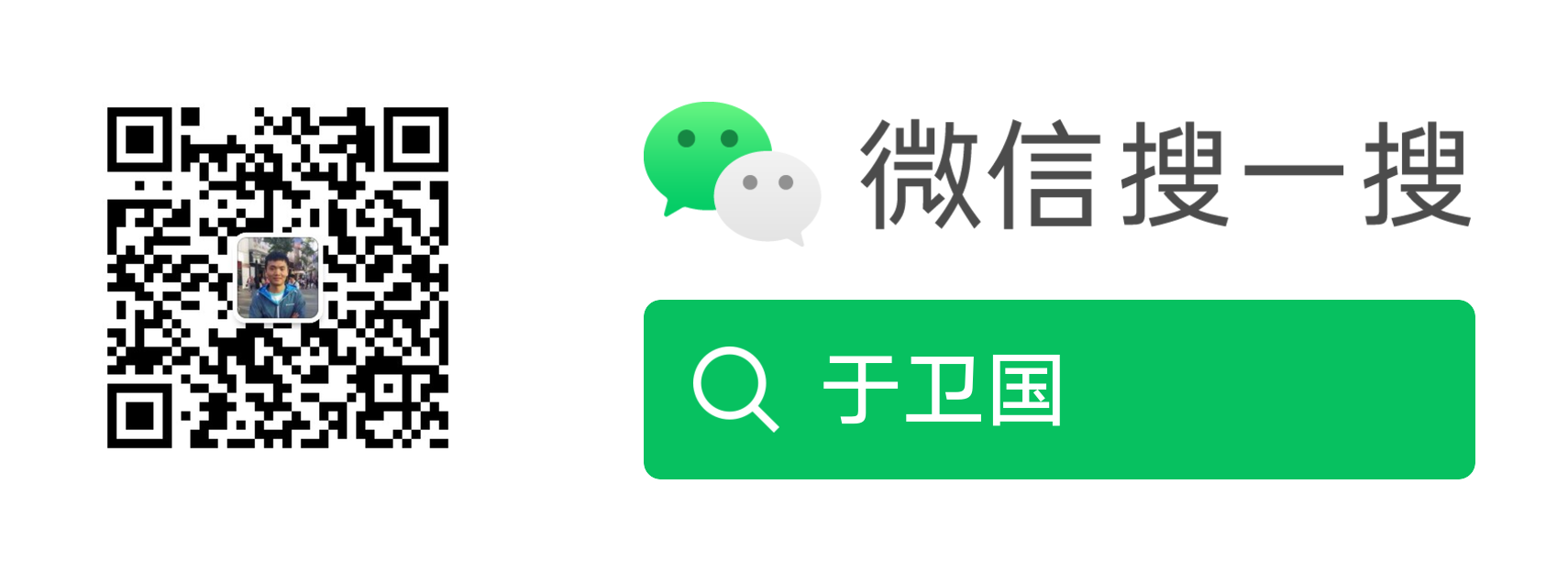